Work with Prism ORM in Nextjs
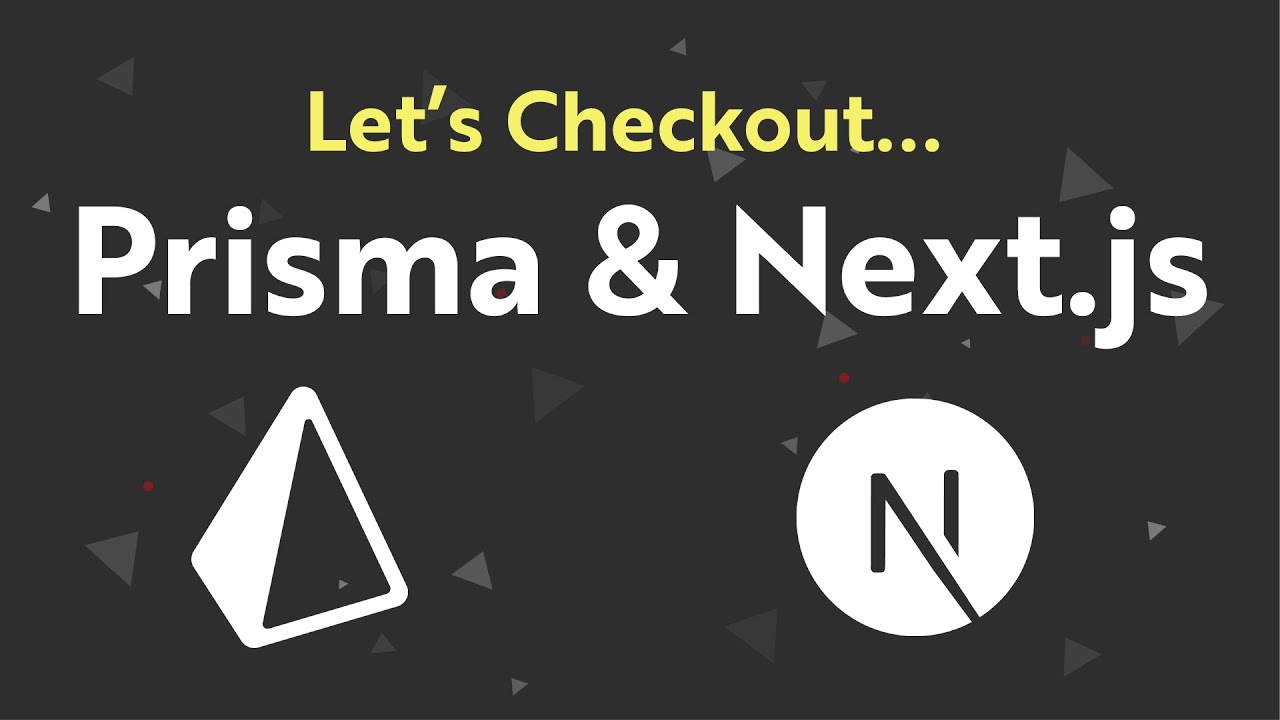
How to quickly and easily setup Prism ORM with Database in Nextjs
Prisma is a ORM for JavaScript and Typescript, it let developers easily configure, create / migrate databases using models. One of the cool feature I love mostly is that , it can be configured with few CLI commands like init, migrate
Setup Prisma
For initializing the Prisma install the developer dependency npm i -d prisma and initialize prisma with
npx prisma init
It will generate necessary files under Prisma folder, please open the file and configure database and models. For demonstration I have configured a Sqlite database, you can use other databases like mysql, postgres, Mongodb etc.
//schema.prisma datasource db { provider = "sqlite" url = "file:./dev.db" } generator client { provider = "prisma-client-js" } model Contact{ id String @id @default(uuid()) name String email String }
Note the id field in the model, it is a primary key and also auto filled by the uuid() function. One you done models go to generate the real database with migrate command
npx prisam migrate dev --name init
This will generate the tables using the models we defined, to make sure we can use the prisma studio which runs on the port 555, also a standalone studio app is available.
In the future we can modify and rerun the migrate command for updating the tables, which will drop the tables and re-create for us.
// run in new terminal npmx prisma studio
Prisma Client
In the nextjs app we need the dependency @prisma/client, let's add them to our project
nmp i -s @prisma/client
In our React file we can now create prisma object and call the fetch method which will get the data from database. Usually we done the fetching with getStaticProps method in React.
//index.js export async function getStaticProps() { const contacts = await prisma.contact.findMany(); return { props: { initialContacts: contacts, }, }; } export default function Home({initialContacts}) { const [contacts, setcontacts] = useState(initialContacts); ....
In the similar way, Prisma can use be used in API or graphql end points too.
Comments